Amaranthine Voyage: The Obsidian Book Collector's Edition
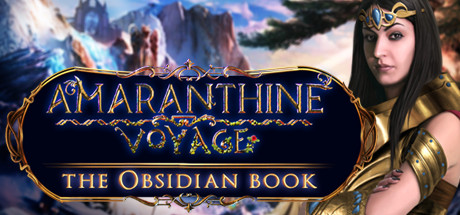
![]() | ... | User Reviews | Too few reviews to generate score |
---|---|---|---|
✽ | 0 | Bundle Count | |
$ | 9.99 | Retail Price | SteamDB, ITAD |
📅 | 2018-07-20 | Release Date | 7 years ago |
Developer | Eipix Entertainment | ||
Publisher | Big Fish Games |
Barter.vg tracks your digital game libraries, wishlists and tradables from different stores. You can find users with the games you want and if they've wishlisted your tradable games.
Sign in through Steam to view the users' collections and trades.
⇄ Tradable 0
★ Wishlist 11 (2%)
📚 Library 11 (2%)
🏷 Tags 5
💻︎ Sites
IsThereAnyDeal current and historic deals
SteamDB Profile the technical details
Steam Trades find trades [Want] or [Have]
Lestrades Profile instant game matching
Powered by Steam store page
Official Site by developer or publisher